Taming Font Size Chaos in React Native: The My11Circle Solution
.webp)
In mobile app development with React Native, dealing with WebView can often present unique challenges. One common issue developers face is the unintended scaling of font sizes within a WebView when the device's font size settings are changed. This can lead to a poor user experience and inconsistent UI across different devices. In this blog post, we'll explore the problem in detail and provide a comprehensive solution to maintain consistent font sizes within both React Native components and the WebView, regardless of the device's settings. We’ll use the My11Circle app as an example to illustrate these issues and solutions.
Understanding the Problem in React Native
When using text components like Text
and TextInput
in a React Native application, changing the device's font size settings affects the font size of these components. This behavior can lead to inconsistencies in the UI, where text elements appear either too large or too small based on the user's device settings. This issue can disrupt the design, making the app look unpolished and less professional.
Real-World Example : My11Circle
Imagine the My11Circle app, a popular fantasy sports platform. The app has a carefully crafted UI with specific font sizes for headings, subheadings, and body text to ensure readability and aesthetic appeal. When a user changes their device's font size settings, these text elements can scale up or down, causing misalignment and affecting the overall readability and aesthetic of the app.
For instance:
Headings: 24px
Subheadings: 18px
Body text: 14px
When a user increases their device's font size settings, the body text might jump to 18px or more, while headings and subheadings also increase disproportionately. This scaling can cause the UI to look cluttered and hard to read, disrupting the user experience.

Understanding the Problem in React Native WebView
The WebView component in React Native, used to display web content within a mobile app, also faces a similar issue. When the device's font size settings are changed, the font sizes within the WebView adjust accordingly. This can be particularly problematic for apps like My11Circle that rely heavily on displaying HTML content or web pages, as the text size changes can disrupt the layout and readability of the content.
Real-World Example: My11Circle
Consider My11Circle’s WebView feature that displays various HTML content, such as player statistics, match previews, or in-depth analysis articles. When the device's font size settings are altered, the text within the WebView might become either too large or too small, making it difficult for users to read the content comfortably.
Solution
To address these issues, we need to ensure that the font sizes within both React Native components and the WebView
remain unaffected by the device's font size settings. Here are the fixes applied:
Fixes for React Native
First, we need to ensure that all text components in the React Native part of the app are not affected by the device's font scaling. This can be achieved by setting the allowFontScaling
property to false for both Text
and TextInput
components. This can be done globally within the componentDidMount
lifecycle method to ensure it applies to all instances of these components throughout the app.
Implementation
Add the following code in the componentDidMount
lifecycle method:
Unset
componentDidMount() {
if (Text.defaultProps == null) {
Text.defaultProps = {};
}
Text.defaultProps.allowFontScaling = false;
TextInput.defaultProps = TextInput.defaultProps || {};
TextInput.defaultProps.allowFontScaling = false;
}
By setting Text.defaultProps.allowFontScaling
and TextInput.defaultProps.allowFontScaling
to false, we ensure that the text sizes in these components remain consistent, regardless of the device's font settings.
Fixes for WebView in React Native
Next, we need to apply a similar fix to the WebView
component. React Native's WebView component, provided by the react-native-webview package, supports a prop called textZoom
which controls the scaling of the text content within the WebView.
Implementation
Unset
import { WebView } from 'react-native-webview';
<WebView textZoom={100} />
Setting textZoom
to 100 ensures that the text within the WebView is rendered at its default size, unaffected by the device's font size settings.
Consistent Font Family in My11Circle
In addition to maintaining consistent font sizes, it's equally important to ensure that the font family used in the app remains unchanged, regardless of the device's font settings. In the My11Circle app, we achieve this by using custom fonts, which are added to the application in a way that ensures they are not affected by any font changes on the user's device.
Implementation
To use custom fonts in a React Native application, the following steps should be taken:
1. Create a folder named fonts inside the assets directory of your application.
2. Place your custom font files (e.g., .ttf or .otf files) inside this fonts folder.
Next, modify the react-native.config.js file to include the fonts in the application:
unset
module.exports = {
project: {
ios: {},
android: {},
},
assets: ['./assets/fonts'],
};
This configuration ensures that your custom fonts are correctly linked and used throughout the My11Circle app, maintaining the desired font family across different devices, regardless of the device's font settings.
Visual Comparison
To illustrate the impact of these fixes, consider the following before and after scenarios using the My11Circle app:
Before
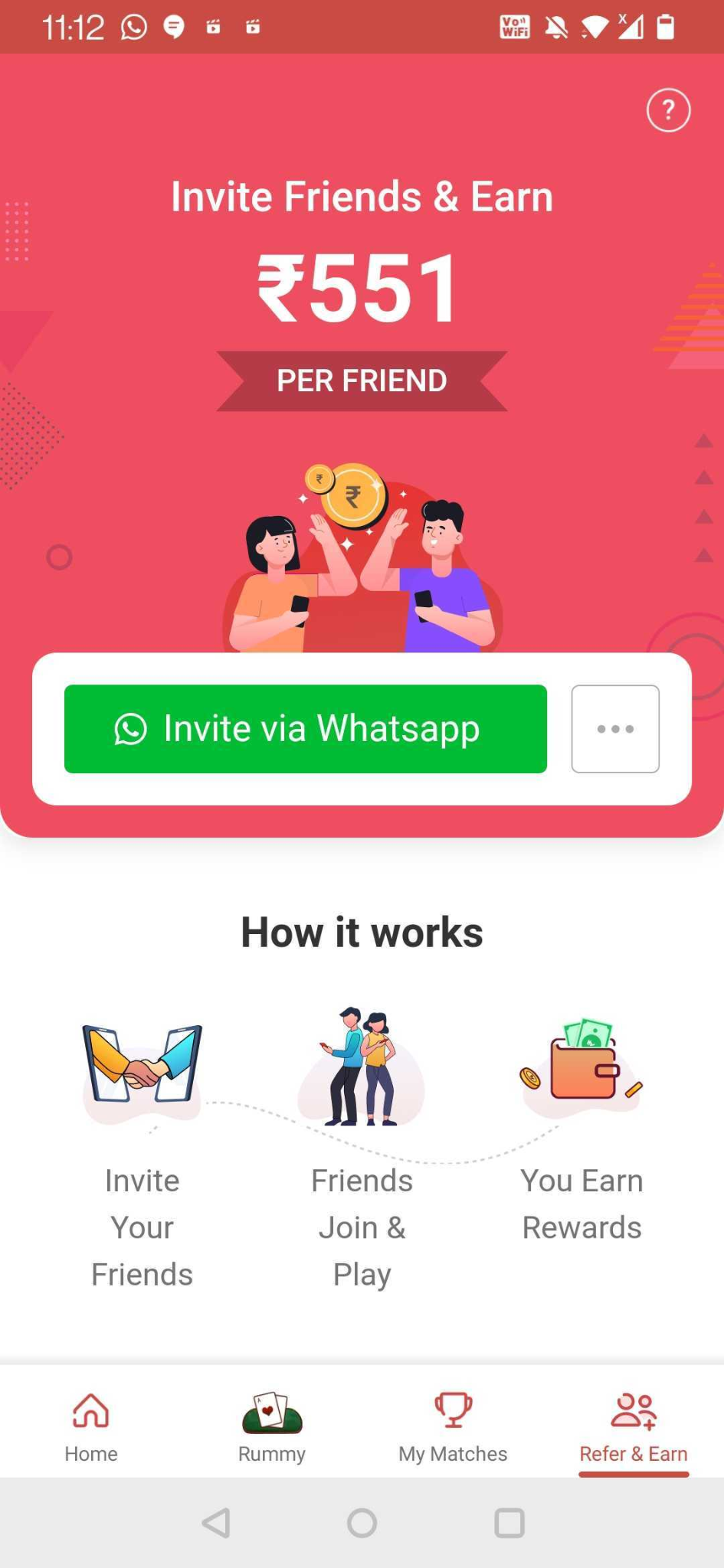
After

Before
In the "before" scenario, the text size within the WebView changes with the device's font settings, leading to inconsistent and unpredictable UI.
Headings: Originally 24px, now scaled to 30px.
Subheadings: Originally 18px, now scaled to 22px.
Body text: Originally 14px, now scaled to 18px.
This scaling causes misalignment and makes the app look cluttered, affecting the user experience negatively.
After
In the "after" scenario, the text size within the WebView remains consistent, providing a stable and predictable user experience.
Headings: Remain at 24px.
Subheadings: Remain at 18px.
Body text: Remain at 14px.
This consistency ensures that the UI looks polished and professional, enhancing the user experience in the My11Circle app.
Comprehensive Solution Implementation
Here’s a step-by-step guide to implementing the fixes in your React Native application:
Step 1: Update Text and TextInput Components
Ensure all Text and TextInput components in your application are unaffected by device font scaling by adding the following code to your main component (e.g., App.js):
import React, { useEffect } from 'react';
import { Text, TextInput } from 'react-native';
const App = () => {
useEffect(() => {
if (Text.defaultProps == null) {
Text.defaultProps = {};
}
Text.defaultProps.allowFontScaling = false;
TextInput.defaultProps = TextInput.defaultProps || {};
TextInput.defaultProps.allowFontScaling = false;
}, []);
return (
// Your app components here
);
};
export default App;
This code ensures that all Text and TextInput components in your app will not scale with the device's font size settings.
Step 2: Configure WebView
Import the WebView component and set the textZoom prop to 100 in the components where you use WebView:
import React from 'react';
import { WebView } from 'react-native-webview';
const WebViewExample = () => {
return (
<WebView
source={{ uri: 'https://example.com' }}
textZoom={100}
/>
);
};
export default WebViewExample;
By setting textZoom to 100, you ensure that the text within the WebView remains at its default size.
Conclusion
By implementing these fixes, you can ensure that the font sizes within both your React Native components and WebView remain consistent and unaffected by the device's font size settings. This approach helps in maintaining a uniform appearance and readability of the content across different devices, enhancing the overall user experience.
Consistent font sizes are crucial for applications that display a significant amount of text, such as the Dream11 app, as they contribute to a polished and professional look, making your application more accessible and user-friendly. If you're facing similar issues in your React Native app, try out these solutions and see the improvement in your text rendering.
Final Thoughts
Addressing font size and font family inconsistencies in React Native and React Native WebView is essential for delivering a seamless user experience. By taking the steps outlined in this post, you can ensure that your app's UI remains consistent and professional across different devices and settings. Consistency not only improves the visual appeal of your app but also enhances usability, making it easier for users to interact with your.
Happy coding!
Author -
Vishnu Khule is a Deputy Manager in UI Development at Play Games24x7 Pvt Ltd, with over 10 years of experience in the industry. He has a strong background in creating and managing user interfaces for various gaming applications. His expertise lies in delivering visually appealing and highly functional UI designs that enhance user engagement and overall gaming experience. Vishnu handles a team of UI developers and always looks for modular approaches, reusable codes, and app size reduction. He is dedicated to maintaining top-notch quality and innovation in UI development, ensuring seamless and immersive experiences for users.
Co-Author -
Nikhil Toraskar is a Sr. UI Developer at Play Games24x7 Pvt Ltd with over 8 years of industry experience. He specializes in creating user-friendly and visually appealing interfaces, contributing significantly to the development of high-quality gaming applications. Nikhil always strives to achieve his tasks using the latest approaches.
Explore More
Discover the latest insights from the world of Games24x7